A Quick way of experimenting with React StarterKit, Material UI and NextJS
A Quick way of experimenting with QuillJS Editor using React StarterKit, Material UI and NextJS
Experimenting with WYSIWYG editor Quill
To start experimenting quickly we'll use a template. This will speed up our process immensely.
Navigate to GitHub template project and use the template by clicking Open in Codespace
:
GitHub - igorrendulic/nextjs-react-typescript-material-starter-template
Contribute to igorrendulic/nextjs-react-typescript-material-starter-template development by creating an account on GitHub.
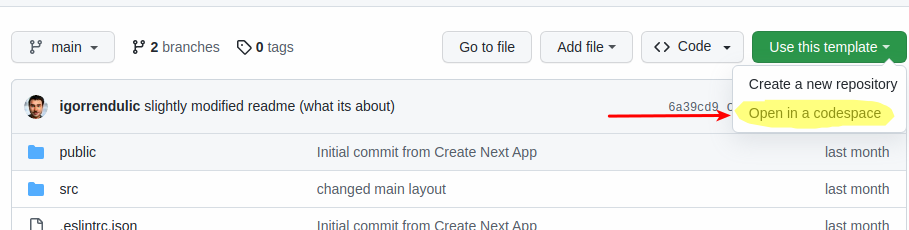
When everything loads go to Terminal and install Quill editor:
yarn add react-quill
Start the server and click Open in Browser
to see our project in the new tab.
yarn dev
We will add quill to home.tsx
component.
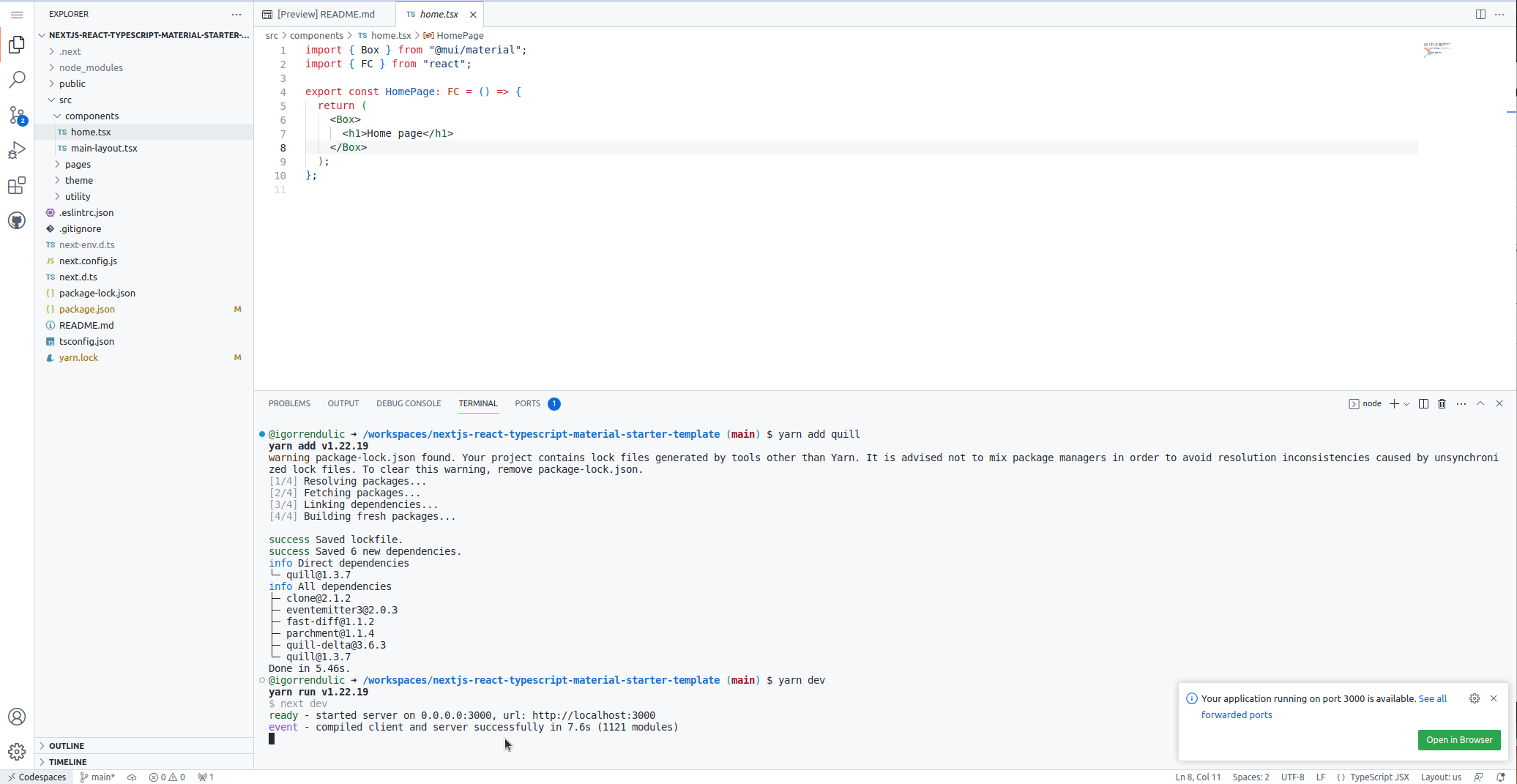
Modify code in home.tsx
:
import { Box } from "@mui/material";
import { FC, useState } from "react";
import 'react-quill/dist/quill.snow.css';
const ReactQuill = typeof window === 'object' ? require('react-quill') : () => false; // SSR problem mitigation
export const HomePage: FC = () => {
const [value, setValue] = useState('');
let modules = {
toolbar: [
[{ 'header': [1, 2, false] }],
['bold', 'italic', 'underline','strike', 'blockquote'],
[{'list': 'ordered'}, {'list': 'bullet'}, {'indent': '-1'}, {'indent': '+1'}],
['link', 'image'],
['clean']
],
};
let formats = [
'header',
'bold', 'italic', 'underline', 'strike', 'blockquote',
'list', 'bullet', 'indent',
'link', 'image'
];
return (
<>
<Box>
<h1>Testing react-quill editor</h1>
</Box>
<Box>
<ReactQuill theme="snow" value={value} modules={modules} formats={formats} onChange={setValue}></ReactQuill>
</Box>
</>
);
};
This is it, check the results in the server tab:
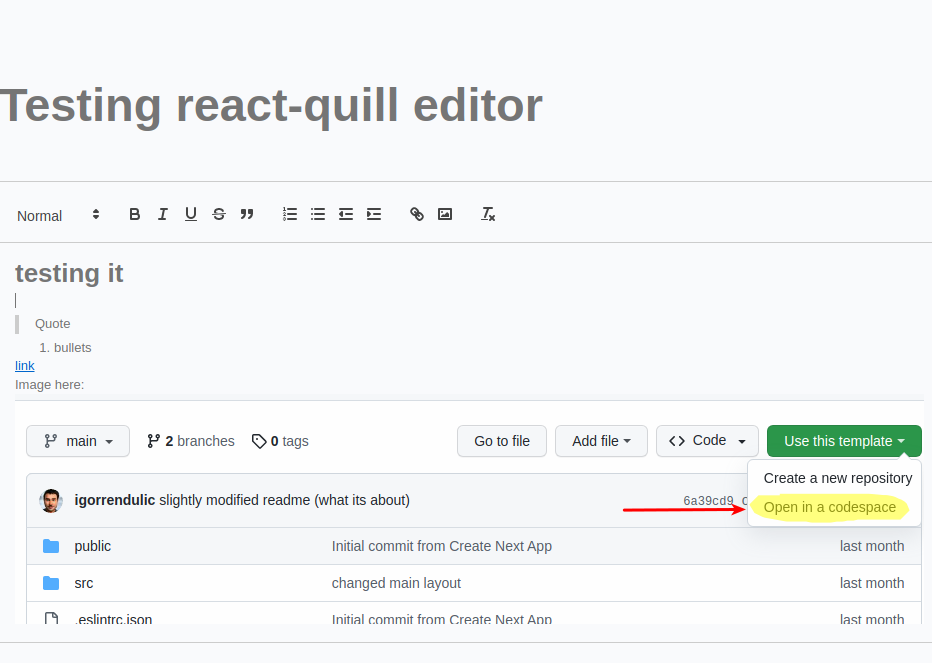